class mrpt::gui::CDisplayWindowGUI¶
A window with powerful GUI capabilities, via the nanogui library.
You can add a background mrpt::opengl::COpenGLScene object rendered on the background of the entire window by setting an object in field background_scene
, locking its mutex background_scene_mtx
.
Refer to nanogui API docs or MRPT examples for further usage examples. A typical lifecycle of a GUI app with this class might look like:
nanogui::init(); {mrpt::gui::CDisplayWindowGUI win; win.drawAll(); win.setVisible(true); nanogui::mainloop(); } nanogui::shutdown();
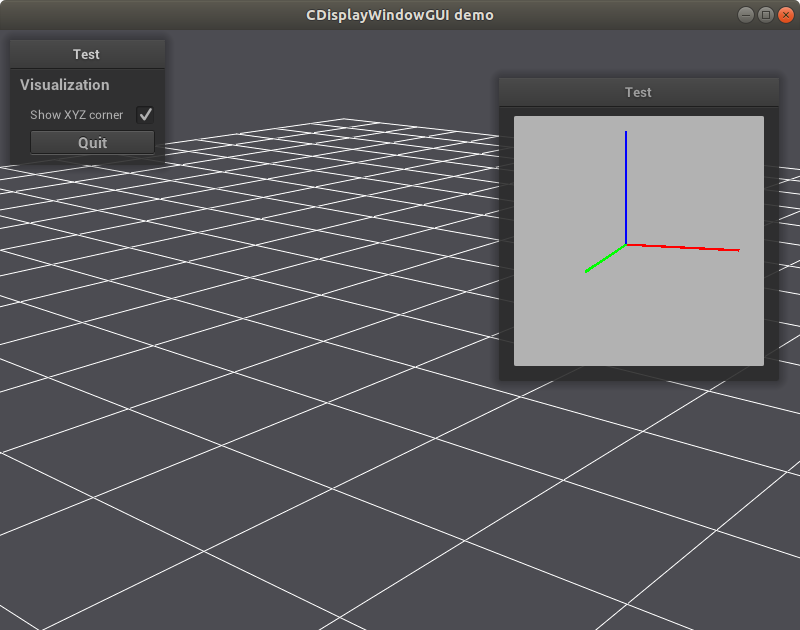
#include <mrpt/gui/CDisplayWindowGUI.h> class CDisplayWindowGUI: public Screen { public: // typedefs typedef std::shared_ptr<CDisplayWindowGUI> Ptr; typedef std::shared_ptr<const CDisplayWindowGUI> ConstPtr; // fields mrpt::opengl::COpenGLScene::Ptr background_scene; std::mutex background_scene_mtx; // construction CDisplayWindowGUI( const std::string& caption = std::string(), unsigned int width = 400, unsigned int height = 300, const CDisplayWindowGUI_Params& p = CDisplayWindowGUI_Params() ); // methods void resize(unsigned int width, unsigned int height); void setPos(int x, int y); void setWindowTitle(const std::string& str); void setLoopCallback(const std::function<void(void)>& callback); const auto& loopCallback() const; void setDropFilesCallback(const std::function<bool(const std::vector<std::string>&)>& callback); const auto& dropFilesCallback() const; void setKeyboardCallback(const std::function<bool(int, int, int, int)>& callback); const auto& keyboardCallback() const; template <typename... Args> static Ptr Create(Args&&... args); CGlCanvasBase& camera(); const CGlCanvasBase& camera() const; nanogui::Window* nanogui_win(); };
Methods¶
void resize(unsigned int width, unsigned int height)
Resizes the window.
void setPos(int x, int y)
Changes the position of the window on the screen.
void setWindowTitle(const std::string& str)
Changes the window title.
void setLoopCallback(const std::function<void(void)>& callback)
Every time the window is about to be repainted, an optional callback can be called, if provided via this method.
void setDropFilesCallback(const std::function<bool(const std::vector<std::string>&)>& callback)
Sets a handle for file drop events.
template <typename... Args> static Ptr Create(Args&&... args)
Class factory returning a smart pointer.