class mrpt::hwdrivers::CGPSInterface¶
A class capable of reading GPS/GNSS/GNSS+IMU receiver data, from a serial port or from any input stream, and parsing the ASCII/binary stream into indivual messages stored in mrpt::obs::CObservationGPS objects.
Typical input streams are serial ports or raw GPS log files. By default, the serial port selected by CGPSInterface::setSerialPortName() or as set in the configuration file will be open upon call to CGenericSensor::initialize(). Alternatively, an external stream can be bound with CGPSInterface::bindStream() before calling CGenericSensor::initialize(). This feature can be used to parse commands from a file, a TCP/IP stream, a memory block, etc.
The parsers in the enum type CGPSInterface::PARSERS are supported as parameter parser
in the configuration file below or in method CGPSInterface::setParser() :
NONE
: Do not try to parse the messages into CObservation’s. Only useful if combined withraw_dump_file_prefix
AUTO
: Try to automatically identify the format of incomming data.NMEA
(NMEA 0183, ASCII messages): Default parser. Supported frames: GGA, RMC,… See full list of messages in children of mrpt::obs::gnss::gnss_messageNOVATEL_OEM6
(Novatel OEM6, binary frames): Supported frames: BESTPOS,… Note that receiving a correct IONUTC msg is required for a correct timestamping of subsequent frames. See full list of messages in children of mrpt::obs::gnss::gnss_message
See available parameters below, and an example config file for rawlog-grabber here
PARAMETERS IN THE ".INI"-LIKE CONFIGURATION STRINGS: ------------------------------------------------------- [supplied_section_name] # Serial port configuration: COM_port_WIN = COM3 COM_port_LIN = ttyUSB0 baudRate = 4800 // The baudrate of the communications (typ. 4800 or 9600 bauds) # (Default:true) Whether to append the GNNS message type to CObservation `sensorLabel` field sensor_label_append_msg_type = true # Select a parser for GNSS data: # Up-to-date list of supported parsers available in https://reference.mrpt.org/devel/classmrpt_1_1hwdrivers_1_1_c_g_p_s_interface.html parser = AUTO # If uncommented and non-empty, raw binary/ascii data received from the serial port will be also dumped # into a file named after this prefix, plus date/time and extension `.gps`. #raw_dump_file_prefix = RAWGPS # 3D position (and orientation, for GNSS+IMUs) of the sensed point (antenna phase center) relative to the vehicle/robot frame: pose_x = 0 // (meters) pose_y = 0 pose_z = 0 pose_yaw = 0 // (deg) pose_pitch = 0 pose_roll = 0 # Optional: list of custom commands to be sent to the GNSS receiver to set it up. # An arbitrary number of commands can be defined, but their names must be "setup_cmd%d" starting at "1". # Commands will be sent by index order. Binary commands instead of ASCII strings can be set programmatically, not from a config file. # custom_cmds_delay = 0.1 // (Default=0.1) Delay in seconds between consecutive set-up commands # custom_cmds_append_CRLF = true // (Default:true) Append "\r\n" to each command # setup_cmd1 = XXXXX # setup_cmd2 = XXXXX # setup_cmd3 = XXXXX # Optional: list of commands to be sent upon disconnection (e.g. object destructor) # shutdown_cmd1 = XXXX # shutdown_cmd2 = XXXX
Note that the customInit
field, supported in MRPT <1.4.0 will be still parsed and obeyed, but since it has been superseded by the new mechanism to establish set-up commands, it is no further documented here.
The next picture summarizes existing MRPT classes related to GPS / GNSS devices (CGPSInterface, CNTRIPEmitter, CGPS_NTRIP):
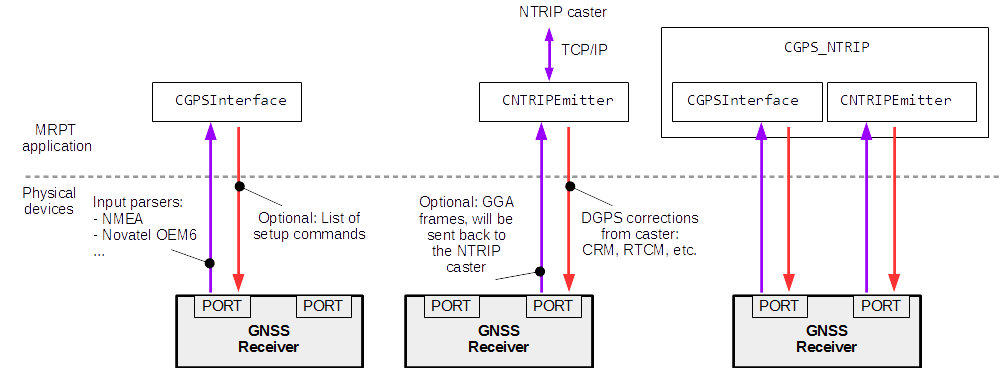
VERSIONS HISTORY:
09/JUN/2006: First version (JLBC)
04/JUN/2008: Added virtual methods for device-specific initialization commands.
10/JUN/2008: Converted into CGenericSensor class (there are no inhirited classes anymore).
07/DEC/2012: Added public static method to parse NMEA strings.
17/JUN/2014: Added GGA feedback.
01/FEB/2016: API changed for MTPT 1.4.0
Verbose debug info will be dumped to cout if the environment variable “MRPT_HWDRIVERS_VERBOSE” is set to “1”, or if you call CGenericSensor::enableVerbose(true)
[API changed in MRPT 1.4.0] mrpt::hwdrivers::CGPSInterface API clean-up and made more generic so any stream can be used to parse GNSS messages, not only serial ports.
See also:
CGPS_NTRIP, CNTRIPEmitter, mrpt::obs::CObservationGPS
#include <mrpt/hwdrivers/CGPSInterface.h> class CGPSInterface: public mrpt::system::COutputLogger, public mrpt::hwdrivers::CGenericSensor { public: // typedefs typedef bool(CGPSInterface::*)(size_t&out_minimum_rx_buf_to_decide) ptr_parser_t; // enums enum PARSERS; // methods void setSerialPortName(const std::string& COM_port); std::string getSerialPortName() const; void setParser(PARSERS parser); PARSERS getParser() const; void bindStream( const std::shared_ptr<mrpt::io::CStream>& external_stream, const std::shared_ptr<std::mutex>& csOptionalExternalStream = std::shared_ptr<std::mutex>() ); bool useExternalStream() const; void setSetupCommandsDelay(const double delay_secs); double getSetupCommandsDelay() const; void setSetupCommands(const std::vector<std::string>& cmds); const std::vector<std::string>& getSetupCommands() const; void setShutdownCommands(const std::vector<std::string>& cmds); const std::vector<std::string>& getShutdownCommands() const; void enableSetupCommandsAppendCRLF(const bool enable); bool isEnabledSetupCommandsAppendCRLF() const; void enableAppendMsgTypeToSensorLabel(bool enable); void setRawDumpFilePrefix(const std::string& filePrefix); std::string getRawDumpFilePrefix() const; bool sendCustomCommand(const void* data, const size_t datalen); bool implement_parser_NMEA(size_t& out_minimum_rx_buf_to_decide); bool implement_parser_NOVATEL_OEM6(size_t& out_minimum_rx_buf_to_decide); virtual void doProcess(); bool isGPS_connected(); bool isGPS_signalAcquired(); bool isAIMConfigured(); std::string getLastGGA(bool reset = true); static bool parse_NMEA(const std::string& cmd_line, mrpt::obs::CObservationGPS& out_obs, const bool verbose = false); };
Inherited Members¶
public: // structs struct TMsg; // methods CGenericSensor& operator = (const CGenericSensor&); virtual void doProcess() = 0;
Methods¶
void setSerialPortName(const std::string& COM_port)
Set the serial port to use (COM1, ttyUSB0, etc).
std::string getSerialPortName() const
Get the serial port to use (COM1, ttyUSB0, etc).
void setParser(PARSERS parser)
Select the parser for incomming data, among the options enumerated in CGPSInterface.
void bindStream( const std::shared_ptr<mrpt::io::CStream>& external_stream, const std::shared_ptr<std::mutex>& csOptionalExternalStream = std::shared_ptr<std::mutex>() )
This enforces the use of a given user stream, instead of trying to open the serial port set in this class parameters.
Call before CGenericSensor::initialize()
Parameters:
csExternalStream |
If not NULL, read/write operations to the stream will be guarded by this critical section. The stream object is not deleted. It is the user responsibility to keep that object allocated during the entire life of this object. |
void setRawDumpFilePrefix(const std::string& filePrefix)
If set to non-empty, RAW GPS serial data will be also dumped to a separate file.
bool sendCustomCommand(const void* data, const size_t datalen)
Send a custom data block to the GNSS device right now.
Can be used to change its behavior online as needed. Can be used to change its behavior online as needed.
Returns:
false on communication error
virtual void doProcess()
This method will be invoked at a minimum rate of “process_rate” (Hz)
Parameters:
This |
method must throw an exception with a descriptive message if some critical error is found. |
bool isGPS_connected()
Returns true if communications work, i.e.
if some message has been received.
bool isGPS_signalAcquired()
Returns true if the last message from the GPS indicates that the signal from sats has been acquired.
std::string getLastGGA(bool reset = true)
Gets the latest GGA command or an empty string if no newer GGA command was received since the last call to this method.
Parameters:
reset |
If set to true, will empty the GGA cache so next calls will return an empty string if no new frame is received. |
static bool parse_NMEA( const std::string& cmd_line, mrpt::obs::CObservationGPS& out_obs, const bool verbose = false )
Parses one line of NMEA data from a GPS receiver, and writes the recognized fields (if any) into an observation object.
Recognized frame types are those listed for the NMEA
parser in the documentation of CGPSInterface
Returns:
true if some new data field has been correctly parsed and inserted into out_obs