class mrpt::obs::CObservationImage
Overview
Declares a class derived from “CObservation” that encapsules an image from a camera, whose relative pose to robot is also stored.
The next figure illustrate the coordinates reference systems involved in this class:
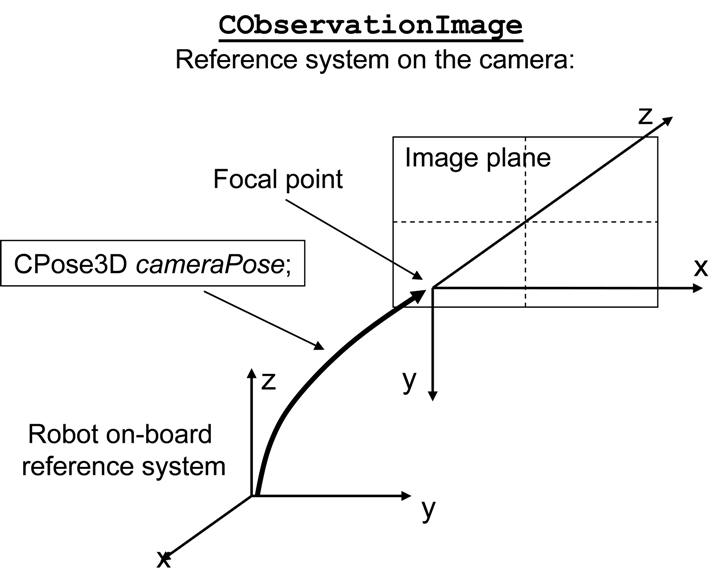
See also:
CObservation, CObservationStereoImages
#include <mrpt/obs/CObservationImage.h> class CObservationImage: public mrpt::obs::CObservation { public: // fields mrpt::poses::CPose3D cameraPose; mrpt::img::TCamera cameraParams; mrpt::img::CImage image; // construction CObservationImage(); // methods virtual void load_impl() const; virtual void unload() const; void getUndistortedImage(mrpt::img::CImage& out_img) const; virtual void getSensorPose(mrpt::poses::CPose3D& out_sensorPose) const; virtual void setSensorPose(const mrpt::poses::CPose3D& newSensorPose); virtual void getDescriptionAsText(std::ostream& o) const; };
Inherited Members
public: // fields mrpt::system::TTimeStamp timestamp {mrpt::Clock::now()}; std::string sensorLabel; // methods mrpt::system::TTimeStamp getTimeStamp() const; virtual mrpt::system::TTimeStamp getOriginalReceivedTimeStamp() const; void load() const; virtual void unload() const; virtual bool exportTxtSupported() const; virtual std::string exportTxtHeader() const; virtual std::string exportTxtDataRow() const; template <class METRICMAP> bool insertObservationInto( METRICMAP& theMap, const std::optional<const mrpt::poses::CPose3D>& robotPose = std::nullopt ) const; virtual void getSensorPose(mrpt::poses::CPose3D& out_sensorPose) const = 0; void getSensorPose(mrpt::math::TPose3D& out_sensorPose) const; mrpt::math::TPose3D sensorPose() const; virtual void setSensorPose(const mrpt::poses::CPose3D& newSensorPose) = 0; void setSensorPose(const mrpt::math::TPose3D& newSensorPose); virtual void getDescriptionAsText(std::ostream& o) const; virtual std::string asString() const;
Fields
mrpt::poses::CPose3D cameraPose
The pose of the camera on the robot.
mrpt::img::TCamera cameraParams
Intrinsic and distortion parameters of the camera.
See the tutorial for a discussion of these parameters.
mrpt::img::CImage image
The image captured by the camera, that is, the main piece of information of this observation.
Construction
CObservationImage()
Constructor.
Parameters:
iplImage |
An OpenCV “IplImage*” object with the image to be loaded in the member “image”, or nullptr (default) for an empty image. |
Methods
virtual void load_impl() const
Makes sure the image, which may be externally stored, are loaded in memory.
See also:
virtual void unload() const
Unload image, for the case of it being stored in lazy-load mode (othewise, the method has no effect).
See also:
void getUndistortedImage(mrpt::img::CImage& out_img) const
Computes the un-distorted image, using the embeded camera intrinsic & distortion parameters.
virtual void getSensorPose(mrpt::poses::CPose3D& out_sensorPose) const
A general method to retrieve the sensor pose on the robot.
Note that most sensors will return a full (6D) CPose3D, but see the derived classes for more details or special cases.
See also:
virtual void setSensorPose(const mrpt::poses::CPose3D& newSensorPose)
A general method to change the sensor pose on the robot.
Note that most sensors will use the full (6D) CPose3D, but see the derived classes for more details or special cases.
See also:
virtual void getDescriptionAsText(std::ostream& o) const
Build a detailed, multi-line textual description of the observation contents and dump it to the output stream.
If overried by derived classes, call base CObservation::getDescriptionAsText() first to show common information.
This is the text that appears in RawLogViewer when selecting an object in the dataset