class mrpt::opengl::CBox
Overview
A solid or wireframe box in 3D, defined by 6 rectangular faces parallel to the planes X, Y and Z (note that the object can be translated and rotated afterwards as any other CRenderizable object using the “object pose” in the base class).
Three drawing modes are possible:
Wireframe: setWireframe(true). Used color is the CRenderizable color
Solid box: setWireframe(false). Used color is the CRenderizable color
Solid box with border: setWireframe(false) + enableBoxBorder(true). Solid color is the CRenderizable color, border line can be set with setBoxBorderColor().
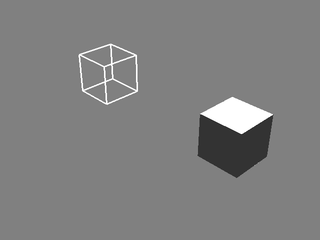
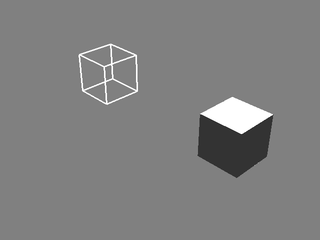
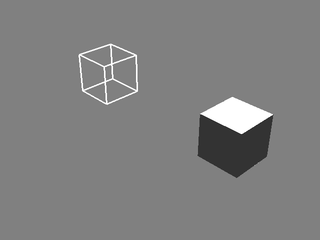
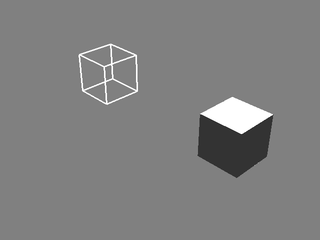
See also:
opengl::Scene, opengl::CRenderizable
#include <mrpt/opengl/CBox.h> class CBox: public mrpt::opengl::CRenderizableShaderTriangles, public mrpt::opengl::CRenderizableShaderWireFrame { public: // construction CBox(); CBox( const mrpt::math::TPoint3D& corner1, const mrpt::math::TPoint3D& corner2, bool is_wireframe = false, float lineWidth = 1.0 ); // methods virtual void render(const RenderContext& rc) const; virtual void renderUpdateBuffers() const; virtual shader_list_t requiredShaders() const; virtual void onUpdateBuffers_Wireframe(); virtual void onUpdateBuffers_Triangles(); virtual void freeOpenGLResources(); virtual mrpt::math::TBoundingBoxf internalBoundingBoxLocal() const; virtual bool traceRay(const mrpt::poses::CPose3D& o, double& dist) const; void setLineWidth(float width); float getLineWidth() const; void setWireframe(bool is_wireframe = true); bool isWireframe() const; void enableBoxBorder(bool drawBorder = true); bool isBoxBorderEnabled() const; void setBoxBorderColor(const mrpt::img::TColor& c); mrpt::img::TColor getBoxBorderColor() const; void setBoxCorners(const mrpt::math::TPoint3D& corner1, const mrpt::math::TPoint3D& corner2); void getBoxCorners( mrpt::math::TPoint3D& corner1, mrpt::math::TPoint3D& corner2 ) const; };
Inherited Members
public: // structs struct OutdatedState; struct RenderContext; struct State; // methods virtual void render(const RenderContext& rc) const = 0; virtual void renderUpdateBuffers() const = 0; virtual shader_list_t requiredShaders() const; virtual void freeOpenGLResources() = 0; virtual void onUpdateBuffers_Triangles() = 0; virtual void onUpdateBuffers_Wireframe() = 0;
Construction
CBox()
Basic empty constructor.
Set all parameters to default.
CBox( const mrpt::math::TPoint3D& corner1, const mrpt::math::TPoint3D& corner2, bool is_wireframe = false, float lineWidth = 1.0 )
Constructor with all the parameters
Methods
virtual void render(const RenderContext& rc) const
Implements the rendering of 3D objects in each class derived from CRenderizable.
This can be called more than once (one per required shader program) if the object registered several shaders.
See also:
virtual void renderUpdateBuffers() const
Called whenever m_outdatedBuffers is true: used to re-generate OpenGL vertex buffers, etc.
before they are sent for rendering in render()
virtual shader_list_t requiredShaders() const
Returns the ID of the OpenGL shader program required to render this class.
See also:
virtual void onUpdateBuffers_Wireframe()
Must be implemented in derived classes to update the geometric entities to be drawn in “m_*_buffer” fields.
virtual void onUpdateBuffers_Triangles()
Must be implemented in derived classes to update the geometric entities to be drawn in “m_*_buffer” fields.
virtual void freeOpenGLResources()
Free opengl buffers.
virtual mrpt::math::TBoundingBoxf internalBoundingBoxLocal() const
Must be implemented by derived classes to provide the updated bounding box in the object local frame of coordinates.
This will be called only once after each time the derived class reports to notifyChange() that the object geometry changed.
See also:
getBoundingBox(), getBoundingBoxLocal(), getBoundingBoxLocalf()
virtual bool traceRay(const mrpt::poses::CPose3D& o, double& dist) const
Ray tracing.
See also:
void setBoxCorners(const mrpt::math::TPoint3D& corner1, const mrpt::math::TPoint3D& corner2)
Set the position and size of the box, from two corners in 3D.