class mrpt::opengl::CMesh
Overview
A planar (XY) grid where each cell has an associated height and, optionally, a texture map.
A typical usage example would be an elevation map or a 3D model of a terrain.
The height of each cell/pixel is provided via an elevation Z
matrix, where the z coordinate of the grid cell (x,y) is given by Z(x,y)
(not Z(y,x)
!!), that is:
Z column count = number of cells in direction “+y”
Z row count = number of cells in direction “+x”
Since MRPT 2.7.0, the texture can be wrapped over the mesh using setMeshTextureExtension().
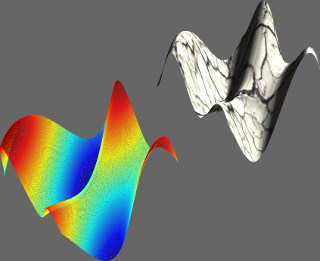
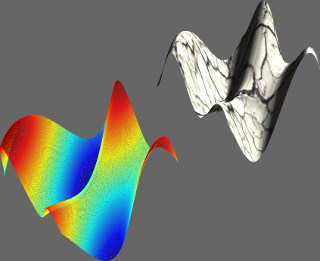
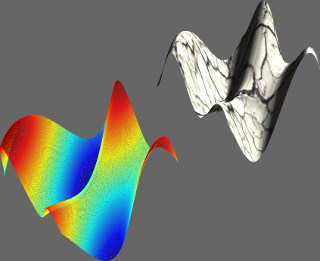
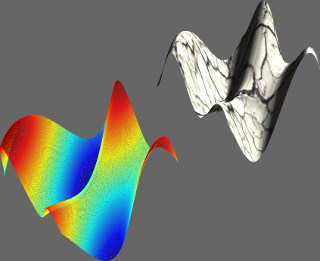
See also:
#include <mrpt/opengl/CMesh.h> class CMesh: public mrpt::opengl::CRenderizableShaderTexturedTriangles, public mrpt::opengl::CRenderizableShaderWireFrame { public: // structs struct TTriangleVertexIndices; // construction CMesh( bool enableTransparency = false, float xMin = -1.0f, float xMax = 1.0f, float yMin = -1.0f, float yMax = 1.0f ); // methods virtual void render(const RenderContext& rc) const; virtual void renderUpdateBuffers() const; virtual shader_list_t requiredShaders() const; virtual void onUpdateBuffers_Wireframe(); virtual void onUpdateBuffers_TexturedTriangles(); virtual void freeOpenGLResources(); template <typename T> void setGridLimits( T xMin, T xMax, T yMin, T yMax ); void getGridLimits( float& xMin, float& xMax, float& yMin, float& yMax ) const; void setMeshTextureExtension(float textureSize_x, float textureSize_y); void getMeshTextureExtension( float& textureSize_x, float& textureSize_y ) const; void enableTransparency(bool v); void enableWireFrame(bool v); void enableColorFromZ( bool v, mrpt::img::TColormap colorMap = mrpt::img::cmHOT ); void setZ(const mrpt::math::CMatrixDynamic<float>& in_Z); void getZ(mrpt::math::CMatrixFloat& out) const; void getMask(mrpt::math::CMatrixFloat& out) const; void setMask(const mrpt::math::CMatrixDynamic<float>& in_mask); float getxMin() const; float getxMax() const; float getyMin() const; float getyMax() const; void setxMin(const float nxm); void setxMax(const float nxm); void setyMin(const float nym); void setyMax(const float nym); void getXBounds( float& min, float& max ) const; void getYBounds( float& min, float& max ) const; void setXBounds( const float min, const float max ); void setYBounds( const float min, const float max ); virtual mrpt::math::TBoundingBoxf internalBoundingBoxLocal() const; void assignImage(const mrpt::img::CImage& img); void assignImageAndZ(const mrpt::img::CImage& img, const mrpt::math::CMatrixDynamic<float>& in_Z); void adjustGridToImageAR(); virtual bool traceRay(const mrpt::poses::CPose3D& o, double& dist) const; void assignImage(const mrpt::img::CImage& img, const mrpt::img::CImage& imgAlpha); void assignImage(mrpt::img::CImage&& img, mrpt::img::CImage&& imgAlpha); void assignImage(mrpt::img::CImage&& img); void cullFaces(const TCullFace& cf); virtual void initializeTextures() const; void enableTextureLinearInterpolation(bool enable); };
Inherited Members
public: // structs struct OutdatedState; struct RenderContext; struct State; // methods virtual void render(const RenderContext& rc) const = 0; virtual void renderUpdateBuffers() const = 0; virtual shader_list_t requiredShaders() const; virtual void freeOpenGLResources() = 0; virtual shader_list_t requiredShaders() const; virtual void render(const RenderContext& rc) const; virtual void renderUpdateBuffers() const; virtual void onUpdateBuffers_TexturedTriangles() = 0; virtual void freeOpenGLResources(); void assignImage(const mrpt::img::CImage& img); virtual void onUpdateBuffers_Wireframe() = 0;
Methods
virtual void render(const RenderContext& rc) const
Implements the rendering of 3D objects in each class derived from CRenderizable.
This can be called more than once (one per required shader program) if the object registered several shaders.
See also:
virtual void renderUpdateBuffers() const
Called whenever m_outdatedBuffers is true: used to re-generate OpenGL vertex buffers, etc.
before they are sent for rendering in render()
virtual shader_list_t requiredShaders() const
Returns the ID of the OpenGL shader program required to render this class.
See also:
virtual void onUpdateBuffers_Wireframe()
Must be implemented in derived classes to update the geometric entities to be drawn in “m_*_buffer” fields.
virtual void onUpdateBuffers_TexturedTriangles()
Must be implemented in derived classes to update the geometric entities to be drawn in “m_*_buffer” fields.
virtual void freeOpenGLResources()
Free opengl buffers.
void setMeshTextureExtension(float textureSize_x, float textureSize_y)
Sets the texture physical size (in “meters) using to wrap it over the mesh extension.
The default (0) means texture size is equal to whole grid extension.
void setZ(const mrpt::math::CMatrixDynamic<float>& in_Z)
This method sets the matrix of heights for each position (cell) in the mesh grid.
void getZ(mrpt::math::CMatrixFloat& out) const
Returns a reference to the internal Z matrix, allowing changing it efficiently.
void getMask(mrpt::math::CMatrixFloat& out) const
Returns a reference to the internal mask matrix, allowing changing it efficiently.
void setMask(const mrpt::math::CMatrixDynamic<float>& in_mask)
This method sets the boolean mask of valid heights for each position (cell) in the mesh grid.
virtual mrpt::math::TBoundingBoxf internalBoundingBoxLocal() const
Must be implemented by derived classes to provide the updated bounding box in the object local frame of coordinates.
This will be called only once after each time the derived class reports to notifyChange() that the object geometry changed.
See also:
getBoundingBox(), getBoundingBoxLocal(), getBoundingBoxLocalf()
void assignImage(const mrpt::img::CImage& img)
Assigns a texture image.
void assignImageAndZ(const mrpt::img::CImage& img, const mrpt::math::CMatrixDynamic<float>& in_Z)
Assigns a texture image and Z simultaneously, and disable transparency.
void adjustGridToImageAR()
Adjust grid limits according to the image aspect ratio, maintaining the X limits and resizing in the Y direction.
virtual bool traceRay(const mrpt::poses::CPose3D& o, double& dist) const
Trace ray.
void assignImage(const mrpt::img::CImage& img, const mrpt::img::CImage& imgAlpha)
Assigns a texture and a transparency image, and enables transparency (If the images are not 2^N x 2^M, they will be internally filled to its dimensions to be powers of two)
Images are copied, the original ones can be deleted.
void assignImage(mrpt::img::CImage&& img, mrpt::img::CImage&& imgAlpha)
Similar to assignImage, but the passed images are moved in (move semantic).
void assignImage(mrpt::img::CImage&& img)
Similar to assignImage, but with move semantics.
void cullFaces(const TCullFace& cf)
Control whether to render the FRONT, BACK, or BOTH (default) set of faces.
Refer to docs for glCullFace()
virtual void initializeTextures() const
VERY IMPORTANT: If you use a multi-thread application, you MUST call this from the same thread that will later destruct the object in order to the OpenGL texture memory to be correctly deleted.
Calling this method more than once has no effects. If you use one thread, this method will be automatically called when rendering, so there is no need to explicitly call it.
void enableTextureLinearInterpolation(bool enable)
Enable linear interpolation of textures (default=false, use nearest pixel)