class mrpt::opengl::CSkyBox
Overview
A Sky Box: 6 textures that are always rendered at “infinity” to give the impression of the scene to be much larger.
Refer to example Example: opengl_skybox_example
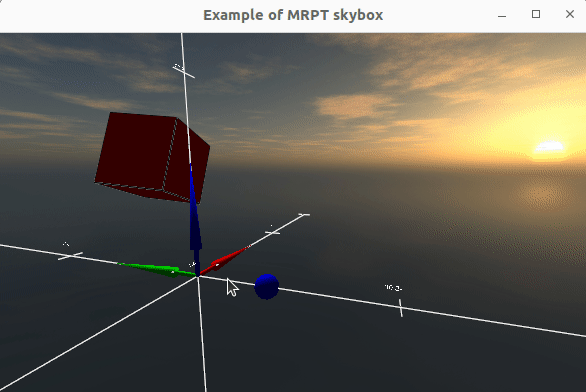
See also:
#include <mrpt/opengl/CSkyBox.h> class CSkyBox: public mrpt::opengl::CRenderizable { public: // construction CSkyBox(); // methods virtual void render(const RenderContext& rc) const; virtual void renderUpdateBuffers() const; virtual shader_list_t requiredShaders() const; virtual void freeOpenGLResources(); virtual void initializeTextures() const; void assignImage(const CUBE_TEXTURE_FACE face, const mrpt::img::CImage& img); void assignImage( const CUBE_TEXTURE_FACE face, img::CImage&& img ); virtual auto internalBoundingBoxLocal() const; virtual bool cullElegible() const; };
Inherited Members
public: // structs struct OutdatedState; struct RenderContext; struct State; // methods virtual void render(const RenderContext& rc) const = 0; virtual void renderUpdateBuffers() const = 0; virtual shader_list_t requiredShaders() const; virtual void freeOpenGLResources() = 0;
Methods
virtual void render(const RenderContext& rc) const
Implements the rendering of 3D objects in each class derived from CRenderizable.
This can be called more than once (one per required shader program) if the object registered several shaders.
See also:
virtual void renderUpdateBuffers() const
Called whenever m_outdatedBuffers is true: used to re-generate OpenGL vertex buffers, etc.
before they are sent for rendering in render()
virtual shader_list_t requiredShaders() const
Returns the ID of the OpenGL shader program required to render this class.
See also:
virtual void freeOpenGLResources()
Free opengl buffers.
virtual void initializeTextures() const
Initializes all textures (loads them into opengl memory).
void assignImage(const CUBE_TEXTURE_FACE face, const mrpt::img::CImage& img)
Assigns a texture.
It is mandatory to assign all 6 faces before initializing/rendering the texture.
Images are copied, the original ones can be deleted.
virtual auto internalBoundingBoxLocal() const
In this class, returns a fixed box (max,max,max), (-max,-max,-max).
virtual bool cullElegible() const
Return false if this object should never be checked for being culled out (=not rendered if its bbox are out of the screen limits).
For example, skyboxes or other special effects.