class mrpt::opengl::CEllipsoidInverseDepth3D
Overview
An especial “ellipsoid” in 3D computed as the uncertainty iso-surfaces of a (inv_range,yaw,pitch) variable.
The parameter space of this ellipsoid comprises these variables (in this order):
inv_range: The inverse distance from the sensor to the feature.
yaw: Angle for the rotation around +Z (“azimuth”).
pitch: Angle for the rotation around +Y (“elevation”). Positive means pointing below the XY plane.
This parameterization is based on the paper:
Civera, J. and Davison, A.J. and Montiel, J., “Inverse depth parametrization for monocular SLAM”, T-RO, 2008.
This class expects you to provide a mean vector of length 3 and a 3x3 covariance matrix, set with setCovMatrixAndMean().
Please read the documentation of CGeneralizedEllipsoidTemplate::setQuantiles() for learning the mathematical details about setting the desired confidence interval.
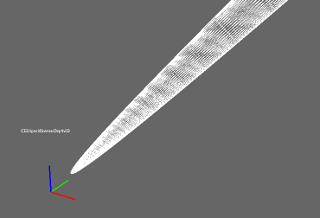
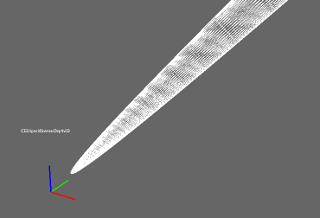
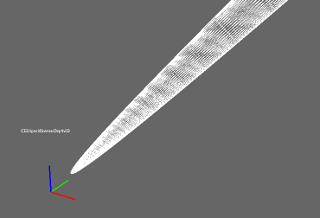
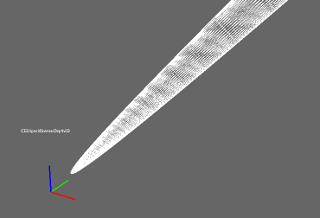
#include <mrpt/opengl/CEllipsoidInverseDepth3D.h> class CEllipsoidInverseDepth3D: public mrpt::opengl::CGeneralizedEllipsoidTemplate, public mrpt::opengl::CRenderizable { public: // construction CEllipsoidInverseDepth3D(); // methods void setUnderflowMaxRange(const float maxRange); float getUnderflowMaxRange() const; };
Inherited Members
public: // structs struct OutdatedState; struct RenderContext; struct State; // methods virtual void render(const RenderContext& rc) const = 0; virtual void renderUpdateBuffers() const = 0; virtual shader_list_t requiredShaders() const; virtual void freeOpenGLResources() = 0; virtual void onUpdateBuffers_Triangles() = 0; virtual void onUpdateBuffers_Wireframe() = 0;
Construction
CEllipsoidInverseDepth3D()
Constructor.
Methods
void setUnderflowMaxRange(const float maxRange)
The maximum range to be used as a correction when a point of the ellipsoid falls in the negative ranges (default: 1e6)