class mrpt::opengl::CColorBar
Overview
A colorbar indicator.
This class renders a colorbar as a 3D object, in the XY plane. For an overlay indicator that can be easily added to any display, see Scene::addColorBar()
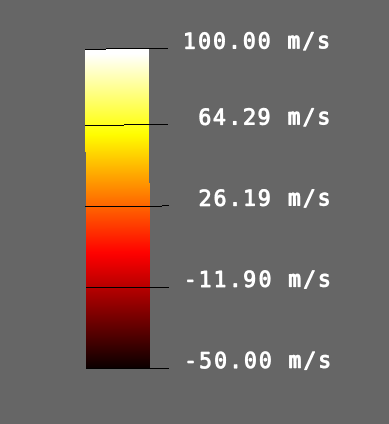
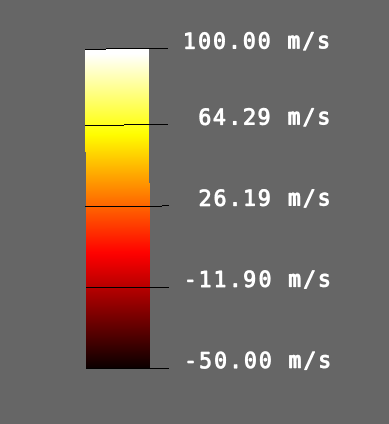
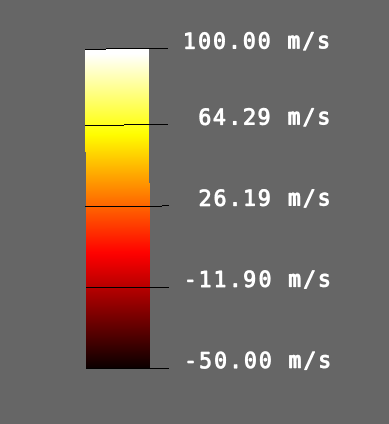
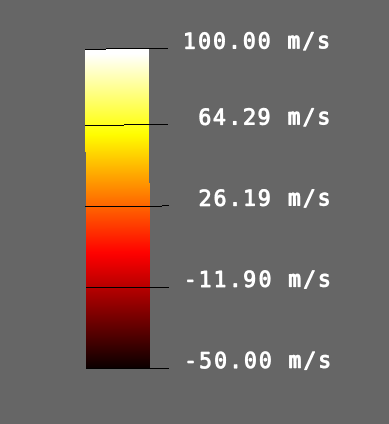
See also:
opengl::Scene, opengl::CRenderizable, Scene::addColorBar()
#include <mrpt/opengl/CColorBar.h> class CColorBar: public mrpt::opengl::CRenderizableShaderTriangles, public mrpt::opengl::CRenderizableShaderWireFrame { public: // construction CColorBar( const mrpt::img::TColormap colormap = mrpt::img::cmGRAYSCALE, double width = 0.2, double height = 1.0, float min_col = .0, float max_col = 1.0, float min_value = .0, float max_value = 1.0, const std::string& label_format = std::string("%7.02f"), float label_font_size = .05f ); // methods virtual void render(const RenderContext& rc) const; virtual void renderUpdateBuffers() const; virtual shader_list_t requiredShaders() const; virtual void onUpdateBuffers_Wireframe(); virtual void onUpdateBuffers_Triangles(); void onUpdateBuffers_all(); virtual void freeOpenGLResources(); virtual mrpt::math::TBoundingBoxf internalBoundingBoxLocal() const; void setColormap(const mrpt::img::TColormap colormap); void setColorAndValueLimits( float col_min, float col_max, float value_min, float value_max ); };
Inherited Members
public: // structs struct OutdatedState; struct RenderContext; struct State; // methods virtual void render(const RenderContext& rc) const = 0; virtual void renderUpdateBuffers() const = 0; virtual shader_list_t requiredShaders() const; virtual void freeOpenGLResources() = 0; virtual void onUpdateBuffers_Triangles() = 0; virtual void onUpdateBuffers_Wireframe() = 0;
Methods
virtual void render(const RenderContext& rc) const
Implements the rendering of 3D objects in each class derived from CRenderizable.
This can be called more than once (one per required shader program) if the object registered several shaders.
See also:
virtual void renderUpdateBuffers() const
Called whenever m_outdatedBuffers is true: used to re-generate OpenGL vertex buffers, etc.
before they are sent for rendering in render()
virtual shader_list_t requiredShaders() const
Returns the ID of the OpenGL shader program required to render this class.
See also:
virtual void onUpdateBuffers_Wireframe()
Must be implemented in derived classes to update the geometric entities to be drawn in “m_*_buffer” fields.
virtual void onUpdateBuffers_Triangles()
Must be implemented in derived classes to update the geometric entities to be drawn in “m_*_buffer” fields.
virtual void freeOpenGLResources()
Free opengl buffers.
virtual mrpt::math::TBoundingBoxf internalBoundingBoxLocal() const
Must be implemented by derived classes to provide the updated bounding box in the object local frame of coordinates.
This will be called only once after each time the derived class reports to notifyChange() that the object geometry changed.
See also:
getBoundingBox(), getBoundingBoxLocal(), getBoundingBoxLocalf()