class mrpt::viz::CArrow
Overview
A 3D arrow.
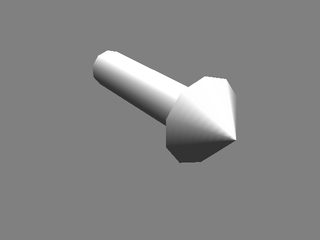
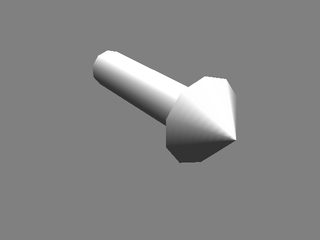
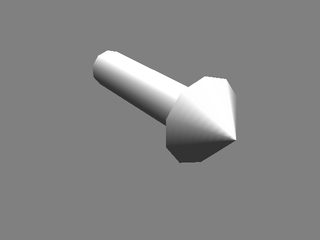
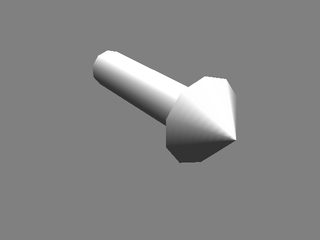
See also:
#include <mrpt/viz/CArrow.h> class CArrow: public mrpt::viz::CVisualObject, public mrpt::viz::VisualObjectParams_Triangles { public: // construction CArrow( float x0 = 0, float y0 = 0, float z0 = 0, float x1 = 1, float y1 = 1, float z1 = 1, float headRatio = 0.2f, float smallRadius = 0.05f, float largeRadius = 0.2f ); CArrow( const mrpt::math::TPoint3Df& from, const mrpt::math::TPoint3Df& to, float headRatio = 0.2f, float smallRadius = 0.05f, float largeRadius = 0.2f ); // methods void setArrowEnds( float x0, float y0, float z0, float x1, float y1, float z1 ); template <typename Vector3Like> void setArrowEnds( const Vector3Like& start, const Vector3Like& end ); void setHeadRatio(float rat); void setSmallRadius(float rat); void setLargeRadius(float rat); void setSlicesCount(uint32_t slices); uint32_t getSlicesCount() const; virtual mrpt::math::TBoundingBoxf internalBoundingBoxLocal() const; virtual bool traceRay(const mrpt::poses::CPose3D& o, double& dist) const; };
Inherited Members
public: // typedefs typedef std::shared_ptr<CObject> Ptr; typedef std::shared_ptr<const CObject> ConstPtr; typedef std::unique_ptr<CObject> UniquePtr; typedef std::unique_ptr<const CObject> ConstUniquePtr; // structs struct OutdatedState; struct State; // methods const auto& shaderTrianglesBuffer() const; auto& shaderTrianglesBufferMutex() const; virtual CVisualObject& setColor_u8(const mrpt::img::TColor& c); bool isLightEnabled() const; void enableLight(bool enable = true); TCullFace cullFaces() const; void notifyBBoxChange() const; auto getBoundingBoxLocalf() const; static const mrpt::rtti::TRuntimeClassId& GetRuntimeClassIdStatic();
Construction
CArrow( float x0 = 0, float y0 = 0, float z0 = 0, float x1 = 1, float y1 = 1, float z1 = 1, float headRatio = 0.2f, float smallRadius = 0.05f, float largeRadius = 0.2f )
Constructor.
Methods
void setSlicesCount(uint32_t slices)
Number of radial divisions
uint32_t getSlicesCount() const
Number of radial divisions
virtual mrpt::math::TBoundingBoxf internalBoundingBoxLocal() const
Must be implemented by derived classes to provide the updated bounding box in the object local frame of coordinates.
This will be called only once after each time the derived class reports to notifyChange() that the object geometry changed.
See also:
getBoundingBox(), getBoundingBoxLocal(), getBoundingBoxLocalf()
virtual bool traceRay(const mrpt::poses::CPose3D& o, double& dist) const
Simulation of ray-trace, given a pose.
Returns true if the ray effectively collisions with the object (returning the distance to the origin of the ray in “dist”), or false in other case. “dist” variable yields undefined behaviour when false is returned