class mrpt::viz::CDisk
Overview
A planar disk in the XY plane.
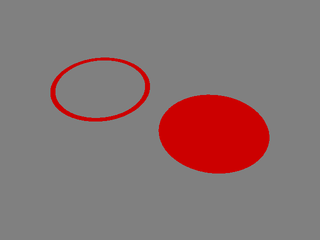
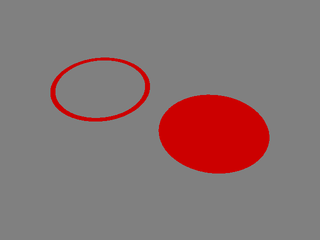
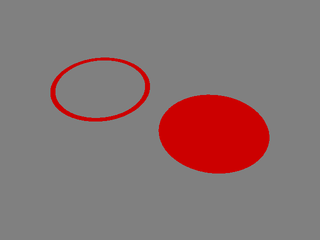
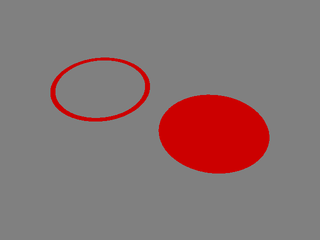
See also:
#include <mrpt/viz/CDisk.h> class CDisk: public mrpt::viz::CVisualObject, public mrpt::viz::VisualObjectParams_Triangles { public: // construction CDisk(); CDisk( float rOut, float rIn, uint32_t slices = 50 ); // methods void setDiskRadius( float outRadius, float inRadius = 0 ); float getInRadius() const; float getOutRadius() const; void setSlicesCount(uint32_t N); virtual mrpt::math::TBoundingBoxf internalBoundingBoxLocal() const; virtual bool traceRay(const mrpt::poses::CPose3D& o, double& dist) const; };
Inherited Members
public: // typedefs typedef std::shared_ptr<CObject> Ptr; typedef std::shared_ptr<const CObject> ConstPtr; typedef std::unique_ptr<CObject> UniquePtr; typedef std::unique_ptr<const CObject> ConstUniquePtr; // structs struct OutdatedState; struct State; // methods const auto& shaderTrianglesBuffer() const; auto& shaderTrianglesBufferMutex() const; virtual CVisualObject& setColor_u8(const mrpt::img::TColor& c); bool isLightEnabled() const; void enableLight(bool enable = true); TCullFace cullFaces() const; void notifyBBoxChange() const; auto getBoundingBoxLocalf() const; static const mrpt::rtti::TRuntimeClassId& GetRuntimeClassIdStatic();
Construction
CDisk()
Constructor.
Methods
void setSlicesCount(uint32_t N)
Default=50.
virtual mrpt::math::TBoundingBoxf internalBoundingBoxLocal() const
Evaluates the bounding box of this object (including possible children) in the coordinate frame of the object parent.
virtual bool traceRay(const mrpt::poses::CPose3D& o, double& dist) const
Ray tracing.