class mrpt::viz::CSetOfLines
Overview
A set of independent lines (or segments), one line with its own start and end positions (X,Y,Z).
Optionally, the vertices can be also shown as dots.
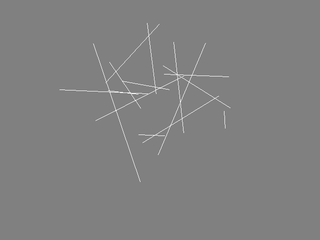
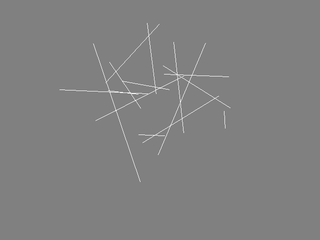
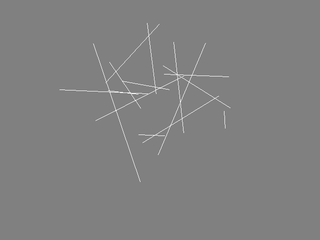
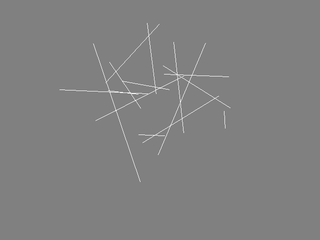
See also:
#include <mrpt/viz/CSetOfLines.h> class CSetOfLines: public mrpt::viz::CVisualObject, public mrpt::viz::VisualObjectParams_Points, public mrpt::viz::VisualObjectParams_Lines { public: // typedefs typedef std::vector<mrpt::math::TSegment3D>::iterator iterator; typedef std::vector<mrpt::math::TSegment3D>::reverse_iterator reverse_iterator; typedef std::vector<mrpt::math::TSegment3D>::const_iterator const_iterator; typedef std::vector<mrpt::math::TSegment3D>::const_reverse_iterator const_reverse_iterator; // construction CSetOfLines(); CSetOfLines(const std::vector<mrpt::math::TSegment3D>& sgms, bool antiAliasing = true); // methods void clear(); float getVerticesPointSize() const; void setVerticesPointSize(const float size_points); void appendLine(const mrpt::math::TSegment3D& sgm); void appendLine(double x0, double y0, double z0, double x1, double y1, double z1); void appendLineStrip(float x, float y, float z); template <class U> void appendLineStrip(const U& point); template <class T> void appendLines(const T& sgms); template <class T_it> void appendLines(const T_it& begin, const T_it& end); void resize(size_t nLines); void reserve(size_t r); template <class T, class U> void appendLine(T p0, U p1); size_t size() const; bool empty() const; void setLineByIndex(size_t index, const mrpt::math::TSegment3D& segm); void setLineByIndex( size_t index, double x0, double y0, double z0, double x1, double y1, double z1 ); void getLineByIndex( size_t index, double& x0, double& y0, double& z0, double& x1, double& y1, double& z1 ) const; const_iterator begin() const; iterator begin(); const_iterator end() const; iterator end(); const_reverse_iterator rbegin() const; const_reverse_iterator rend() const; virtual mrpt::math::TBoundingBoxf internalBoundingBoxLocal() const; };
Inherited Members
public: // structs struct OutdatedState; struct State; // methods const auto& shaderPointsVertexPointBuffer() const; const auto& shaderPointsVertexColorBuffer() const; auto& shaderPointsBuffersMutex() const; float getPointSize() const; bool isEnabledVariablePointSize() const; float getVariablePointSize_k() const; float getVariablePointSize_DepthScale() const; void setLineWidth(float w); float getLineWidth() const; void enableAntiAliasing(bool enable = true); bool isAntiAliasingEnabled() const;
Construction
CSetOfLines()
Constructor.
CSetOfLines(const std::vector<mrpt::math::TSegment3D>& sgms, bool antiAliasing = true)
Constructor with a initial set of lines.
Methods
void clear()
Clear the list of segments.
void setVerticesPointSize(const float size_points)
Enable showing vertices as dots if size_points>0.
void appendLine(const mrpt::math::TSegment3D& sgm)
Appends a line to the set.
void appendLine( double x0, double y0, double z0, double x1, double y1, double z1 )
Appends a line to the set, given the coordinates of its bounds.
void appendLineStrip(float x, float y, float z)
Appends a line whose starting point is the end point of the last line (similar to OpenGL’s GL_LINE_STRIP)
Parameters:
std::exception |
If there is no previous segment |
template <class U> void appendLineStrip(const U& point)
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
template <class T> void appendLines(const T& sgms)
Appends any iterable collection of lines to the set.
Note that this includes another CSetOfLines.
See also:
template <class T_it> void appendLines(const T_it& begin, const T_it& end)
Appends certain amount of lines, located between two iterators, into the set.
See also:
void resize(size_t nLines)
Resizes the set.
See also:
void reserve(size_t r)
Reserves an amount of lines to the set.
This method should be used when some known amount of lines is going to be inserted, so that only a memory allocation is needed.
See also:
template <class T, class U> void appendLine(T p0, U p1)
Inserts a line, given its bounds.
Works with any pair of objects with access to x, y and z members.
size_t size() const
Returns the total count of lines in this set.
bool empty() const
Returns true if there are no line segments.
void setLineByIndex(size_t index, const mrpt::math::TSegment3D& segm)
Sets a specific line in the set, given its index.
See also:
void setLineByIndex( size_t index, double x0, double y0, double z0, double x1, double y1, double z1 )
Sets a specific line in the set, given its index.
See also:
void getLineByIndex( size_t index, double& x0, double& y0, double& z0, double& x1, double& y1, double& z1 ) const
Gets a specific line in the set, given its index.
See also:
const_iterator begin() const
Beginning const iterator.
See also:
const_iterator end() const
Ending const iterator.
See also:
const_reverse_iterator rbegin() const
Beginning const reverse iterator (actually, accesses the end of the set).
See also:
const_reverse_iterator rend() const
Ending const reverse iterator (actually, refers to the starting point of the set).
See also:
virtual mrpt::math::TBoundingBoxf internalBoundingBoxLocal() const
Evaluates the bounding box of this object (including possible children) in the coordinate frame of the object parent.